Even Odd Or Neither Function Calculator
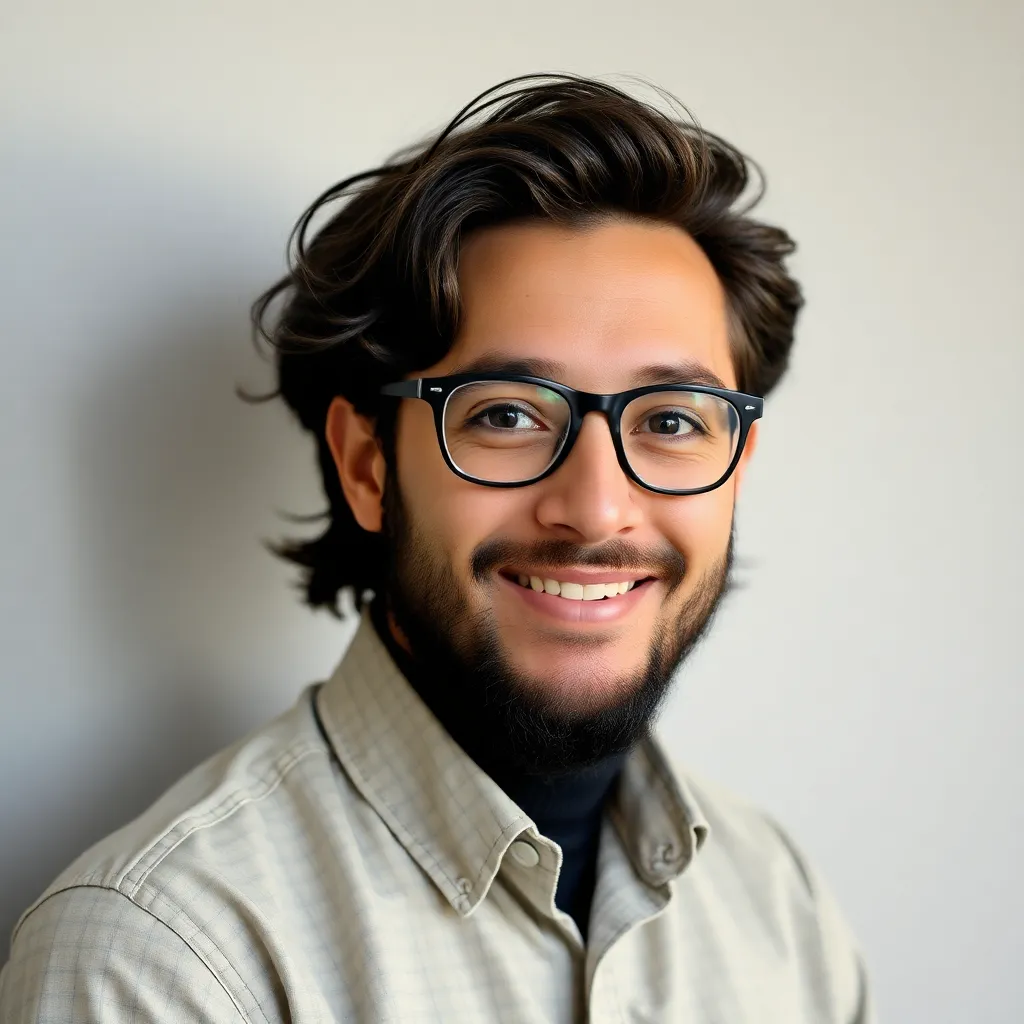
Greels
Mar 28, 2025 · 7 min read
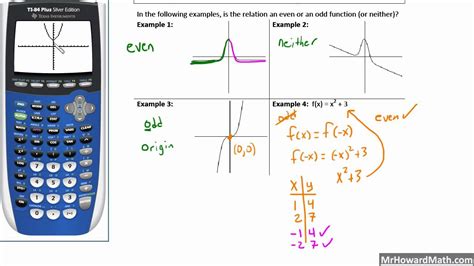
Table of Contents
Even, Odd, or Neither Function Calculator: A Comprehensive Guide
Determining whether a function is even, odd, or neither is a fundamental concept in mathematics, particularly in calculus and advanced algebra. Understanding function parity helps simplify complex problems and offers valuable insights into the symmetry and behavior of mathematical functions. This comprehensive guide will not only explain the concepts of even, odd, and neither functions but also provide a step-by-step approach to building your own Even, Odd, or Neither Function Calculator. We'll explore various programming methods and delve into the practical applications of this crucial mathematical concept.
Understanding Even, Odd, and Neither Functions
Before diving into the creation of a calculator, let's solidify our understanding of the core concepts.
Even Functions
A function f(x) is considered even if it satisfies the following condition:
f(-x) = f(x)
This means that replacing x with -x does not change the value of the function. Graphically, even functions exhibit symmetry about the y-axis. Classic examples include:
- f(x) = x²: (-x)² = x², hence it's even.
- f(x) = cos(x): cos(-x) = cos(x), therefore it's even.
- f(x) = |x|: |-x| = |x|, demonstrating even function behavior.
Odd Functions
A function f(x) is considered odd if it satisfies this condition:
f(-x) = -f(x)
In this case, replacing x with -x results in the negative of the original function's value. Graphically, odd functions exhibit symmetry about the origin (0,0). Examples include:
- f(x) = x³: (-x)³ = -x³, thus it's odd.
- f(x) = sin(x): sin(-x) = -sin(x), illustrating an odd function.
- f(x) = x⁵ - 3x: (-x)⁵ - 3(-x) = -x⁵ + 3x = -(x⁵ - 3x), hence odd.
Neither Even Nor Odd Functions
Many functions do not fall neatly into either the even or odd category. These are classified as neither even nor odd. They lack the symmetrical properties of even and odd functions. Examples are:
- f(x) = x + 1: f(-x) = -x + 1 ≠ f(x) and f(-x) ≠ -f(x).
- f(x) = eˣ: e⁻ˣ ≠ eˣ and e⁻ˣ ≠ -eˣ.
- f(x) = x² + x: This function combines an even term (x²) and an odd term (x), resulting in neither even nor odd behavior.
Building an Even, Odd, or Neither Function Calculator
Now, let's explore how to build a calculator capable of determining the parity of a given function. We'll examine several approaches, focusing on simplicity and clarity.
Method 1: A Simple Algebraic Approach (Conceptual)
This method involves direct substitution and comparison. Let's assume our function is represented as f(x)
. The algorithm would be:
- Input: Obtain the function f(x) from the user (This might involve a string representation of the function or a defined mathematical expression).
- Calculate f(x): Evaluate the function at a chosen value of x.
- Calculate f(-x): Evaluate the function at -x.
- Comparison:
- If
f(-x) = f(x)
, the function is even. - If
f(-x) = -f(x)
, the function is odd. - Otherwise, the function is neither even nor odd.
- If
- Output: Display the result.
Limitations: This approach heavily relies on symbolic manipulation and accurate function evaluation, which can be complex for intricate functions. It's best suited for simpler functions that can be easily handled algebraically.
Method 2: Numerical Approach using a Programming Language (Python Example)
This approach is more robust and suitable for a wider range of functions. We'll use Python for its ease of use and powerful libraries.
def check_function_parity(func, x):
"""
Checks if a function is even, odd, or neither.
Args:
func: The function to check (a callable object).
x: A non-zero value to evaluate the function at.
Returns:
"Even", "Odd", or "Neither".
"""
try:
fx = func(x)
f_minus_x = func(-x)
if abs(f_minus_x - fx) < 1e-6: # Account for floating-point precision errors
return "Even"
elif abs(f_minus_x + fx) < 1e-6:
return "Odd"
else:
return "Neither"
except Exception as e:
return f"Error: {e}"
# Example usage:
def my_function(x):
return x**2 + 2
result = check_function_parity(my_function, 2)
print(f"The function is: {result}")
def my_function2(x):
return x**3
result2 = check_function_parity(my_function2, 2)
print(f"The function is: {result2}")
def my_function3(x):
return x + 1
result3 = check_function_parity(my_function3, 2)
print(f"The function is: {result3}")
This Python code defines a function check_function_parity
that takes the function and a value x
as input. It calculates f(x)
and f(-x)
and compares them to determine the parity, accounting for potential floating-point inaccuracies. The try-except
block gracefully handles potential errors during function evaluation.
Method 3: Graphical Approach (Conceptual)
A graphical approach involves plotting the function and visually inspecting its symmetry.
- Plot: Generate a graph of the function f(x).
- Visual Inspection:
- If the graph is symmetrical about the y-axis, the function is even.
- If the graph is symmetrical about the origin, the function is odd.
- If neither symmetry is observed, the function is neither even nor odd.
Limitations: This method relies on visual interpretation and might be less precise for complex functions or those with subtle symmetry variations. It's a useful method for initial exploration and visualization but not suitable for precise algorithmic determination.
Advanced Considerations and Error Handling
The numerical approach (Method 2) is more practical for a real-world calculator. However, to make it more robust, consider these enhancements:
- Input Validation: Implement input validation to handle invalid function inputs or non-numeric values. This can prevent crashes and improve user experience.
- Error Handling: Include comprehensive error handling to gracefully manage situations like division by zero, invalid function definitions, or other runtime errors.
- Numerical Precision: The example uses a tolerance (
1e-6
) to account for floating-point inaccuracies. Adjust this tolerance as needed for higher precision or different applications. - User Interface: For a user-friendly calculator, incorporate a graphical user interface (GUI) using libraries like Tkinter (Python) or similar GUI frameworks for other languages. This will make the calculator easier to use and more accessible.
- Function Parsing: For a more sophisticated calculator, implement a function parser to handle function input as strings (e.g., "x^2 + 2*x"). This requires a parsing library or custom implementation to translate the string representation into a callable function.
Applications of Even, Odd, and Neither Function Determination
Determining function parity has several significant applications:
- Fourier Series: In signal processing and other areas, the Fourier series decomposes a periodic function into a sum of sine and cosine functions. Knowing whether a function is even or odd simplifies this decomposition.
- Calculus: Function parity simplifies integration and differentiation, especially in symmetric intervals. For example, the integral of an odd function over a symmetric interval is zero.
- Physics and Engineering: Many physical phenomena are described by functions exhibiting even or odd symmetry. For instance, the potential energy in some systems is an even function, while the velocity in others might be odd.
- Linear Algebra: In linear algebra, the concept of even and odd functions relates to the properties of matrices and their transformations.
- Differential Equations: Symmetry considerations arising from function parity can simplify the solution of differential equations.
Conclusion
Determining whether a function is even, odd, or neither is a fundamental concept in mathematics with widespread applications across various fields. While a purely algebraic approach works for simple functions, a robust numerical method, as demonstrated with the Python example, is better suited for building a practical Even, Odd, or Neither Function Calculator. By incorporating error handling, input validation, and potentially a graphical user interface, you can create a powerful and user-friendly tool for mathematical analysis. The advanced considerations discussed here will enhance the reliability and versatility of your calculator, making it a valuable asset for students, researchers, and engineers alike. Remember to continually refine your calculator by incorporating user feedback and expanding its capabilities to handle more complex functions and situations.
Latest Posts
Latest Posts
-
26 2 Miles Is How Many Kilometers
Mar 31, 2025
-
How Many Kg Is 136 Pounds
Mar 31, 2025
-
173 Inches Is How Many Feet
Mar 31, 2025
-
What Is 86 Inches In Cm
Mar 31, 2025
-
5 4x 7 4x 2 X
Mar 31, 2025
Related Post
Thank you for visiting our website which covers about Even Odd Or Neither Function Calculator . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.